
SQL Injection Problem in Hibernate Wth Parameter Binding
In this tutorial of SQL Injection and Parameter Binding in Hibernate we will discuss about SQL inject and its demerits and also describe Parameter binding, it means is way to bind parameter with SQL to use in the hibernate...
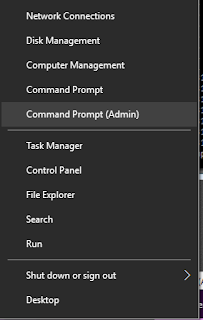
Backup and Restore Windows Drivers without any Software
You can backup all the device drivers installed on your current working system configuration without any software and also restore them if you format the operating system for any reason.Do these simple steps:
Backup Drivers:
1. Run...

Working Of Garbage Collector
In Java, allocation and de-allocation of memory space for objects are done by the garbage collection process in an automated way by the JVM.Java Memory ManagementJava Memory Management, with its built-in garbage collection, is one of the language’s...
Garbage Collector In java
Garbage Collector is part of JRE that makes sure that object that are not referenced will be freed from memory. Garbage collector can be viewed as a reference count manager. if an object is created and its reference is stored in a variable, its reference count is increased by one. during the course of execution...

Create a Web App using Java Servlets
2016-20-11
664
One of nicest features of Java is its rich, multifaceted nature. Sure, building traditional desktop and even mobile applications is all well and fine. But what if you want to leave your current background behind...